Data Structures & Algorithms
Develop a deep understanding of Data Structures & Algorithms. If you feel you learned this enough in college, you can skip this step.
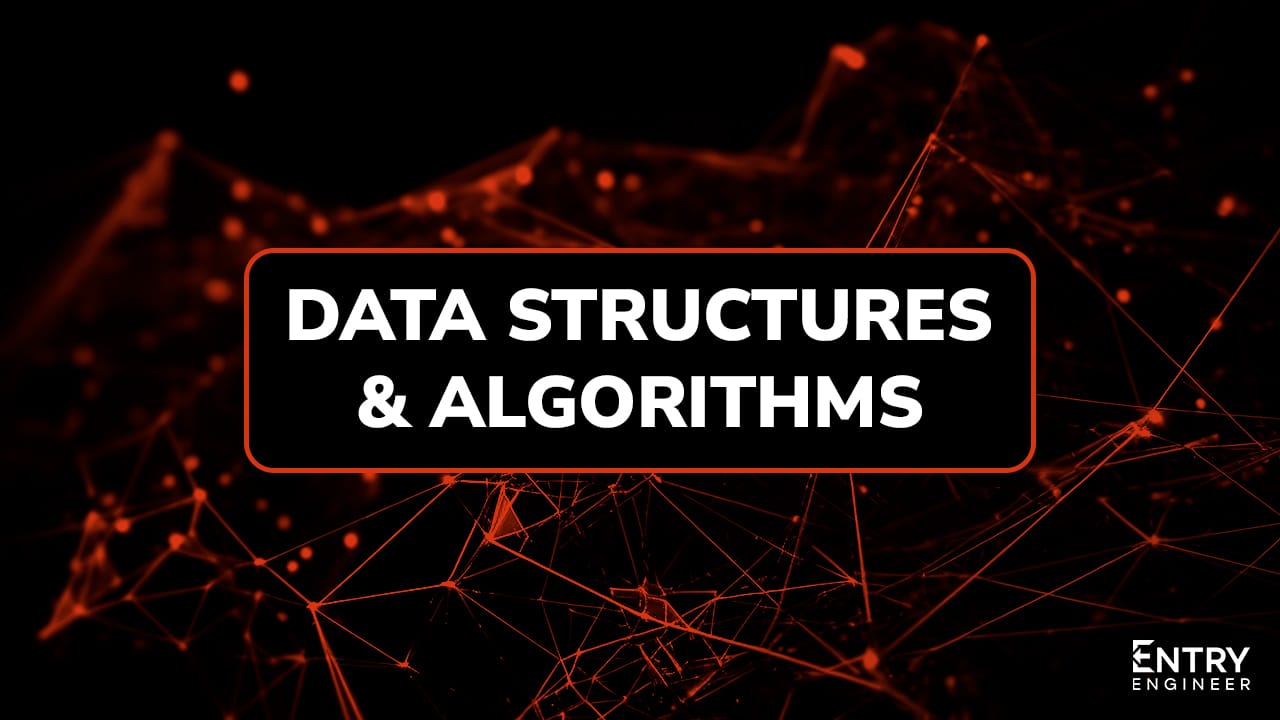
Data Structures & Algorithms:
Imagine you’re trying to build an epic LEGO spaceship, but all the pieces are dumped in a giant pile with no instructions. You’d probably spend forever just finding the right parts, let alone putting them together. That’s what coding can feel like without Data Structures and Algorithms—or DSA for short. They’re like your magic sorting bins and a clear game plan that turn chaos into something awesome. In this guide, I’ll walk you through why DSA is a big deal (especially for landing coding jobs), what it really means in plain English, some cool examples you’ll recognize, and why sticking with it is worth your time.
Why DSA Matters When You’re Trying to Get a Coding Job
Picture this: you’re sitting in a job interview for a cool gig—maybe building apps, working on robots, or crunching data. The interviewer slides a problem your way and says, “Solve this.” Chances are, they’re testing your DSA skills. Why do they care so much? It’s not just to mess with you—it’s because DSA shows how you think and tackle tough stuff. Here’s the scoop:
- It’s Like a Brain Workout: These problems are like riddles. If you can crack them, it proves you’re good at breaking big messes into bite-sized steps. Companies love that because real coding jobs are full of tricky puzzles.
- Speed and Smarts: Picking the right data structure or algorithm can make your code zoom instead of crawl. Big companies deal with mountains of info and millions of users, so they need people who can keep things fast and slick.
- Growing Without Crashing: Ever wonder how apps handle more and more people without falling apart? That’s scalability, and DSA helps you build stuff that can stretch without breaking. Interviewers want to know you’re thinking ahead.
- The Basics That Stick: Once you’ve got DSA down, it’s like knowing the secret sauce of coding. It works everywhere, no matter what tools or languages you use, and it makes picking up new skills a breeze.
Nail a DSA question in an interview, and you’re basically shouting, “I’ve got this!” It’s your chance to show you can think sharp and code smart, even when the pressure’s on.
So, What’s This DSA Thing Anyway?
Don’t let the fancy name scare you—Data Structures and Algorithms just means organizing stuff and figuring out how to use it. Let’s break it down super simple:
- Data Structures: Think of these as clever ways to store your toys—or, in coding, your info. It’s like having shelves, boxes, or bags that keep things neat so you can grab what you need fast. For example, if you’ve got a pile of game cards, a data structure decides whether they’re stacked, lined up, or sorted by type.
- Algorithms: These are your how-to guides. Like when you’re making a sandwich—grab bread, spread peanut butter, add jelly, squish it together—an algorithm is a list of steps to get something done. In coding, it’s the plan for sorting numbers, finding a name, or whatever else you need.
Put them together, and DSA is about keeping your data tidy and knowing exactly what to do with it. It’s like being a chef with a killer kitchen setup and the perfect recipe.
Fun Examples of Data Structures You’ll Get Right Away
Data structures are everywhere, and they’re not as weird as they sound. Here are some you’ve probably seen in real life:
- Arrays: Imagine a row of cubbyholes, like a shoe rack. Each slot’s got a number, and you can pop something in or pull it out quick. In coding, arrays hold stuff in order—like a list of your top five video game scores—so you can jump straight to the third one without digging.
- Linked Lists: Picture a treasure hunt where each clue points to the next spot. That’s a linked list—each piece of data is tied to the one after it. Want to add something? Just hook it in. It’s perfect when you don’t know how much stuff you’ll end up with.
- Stacks: Ever stack books or pancakes? You pile them up, and when you need one, you grab the top. That’s a stack—last thing in, first thing out. It’s awesome for things like hitting “undo” in a game or app.
- Queues: Think of waiting in line for ice cream. First person there gets served first, and new folks join the back. That’s a queue—first in, first out. It’s how your printer knows which page to spit out next.
- Hash Tables: Imagine a notebook where you write someone’s name and their phone number next to it. Later, you flip to their name and—bam—there’s the number. Hash tables are like that: give it a key (the name), and it hands you the info (the number) lightning-fast.
- Trees: Ever draw a family tree? Start with Grandma at the top, then branch out to parents and kids. Trees in coding work the same way, organizing stuff with a top spot (the root) and branches below—like folders on your computer splitting into more folders.
Each one’s got its own vibe, and picking the right structure makes coding way easier.
Algorithms: The Plans That Make Things Happen
Now, let’s talk algorithms—the step-by-step moves that get stuff done. Here are a few you’ll run into:
- Sorting (Bubble Sort and Quick Sort):
- Bubble Sort: Imagine lining up your friends by height. You check two at a time—if the taller one’s in front, they swap. Keep going until everyone’s in place. It’s simple but takes a while if the group’s big.
- Quick Sort: This one’s slicker. Pick a height, then split your friends into “shorter” and “taller” groups around it. Keep splitting those groups the same way until they’re all sorted. It’s faster because it chops the work into chunks.
- Searching (Binary Search): Say you’re guessing a number between 1 and 100. Start at 50. Too high? Try 25. Too low? Go to 75. Each guess cuts your options in half, so you find it quick—but only if the list’s already sorted.
- Pathfinding (Dijkstra’s Algorithm): Think of this as your phone’s map app. Starting at home, it checks every road, always trying the shortest one next, until it finds the fastest way to school. It’s how GPS figures out your quickest route.
These are like your playbook—knowing them means you’ve got a move for every challenge.
Why Bother Learning DSA?
Sure, DSA helps you ace interviews, but it’s bigger than that. It’s like unlocking a superpower for coding that sticks with you forever. Here’s why it’s worth the effort:
- Faster, Smarter Code: With DSA, you’ll spot when your program’s dragging and fix it—like swapping a slow list for a zippy hash table. It’s a must when you’re dealing with tons of info.
- Problem-Solving Skills: Wrestling with algorithms sharpens your brain. You’ll get better at untangling messes, whether you’re fixing bugs or dreaming up new ideas.
- Building Stuff That Grows: Want your app to handle a million users? DSA helps you plan ahead so it doesn’t crash when things get huge.
- Skills That Last: Once you’ve got DSA, it works anywhere. New language? No problem. It’s like learning to ride a bike—you don’t forget, and it takes you places.
It’s not just about passing a test; it’s about becoming a coder who can handle anything.
How to Jump Into DSA Without Freaking Out
Ready to start? Don’t worry if it feels big—everyone begins at zero. Here’s how to make it fun and doable:
- Keep It Simple: Kick off with easy stuff like arrays or sorting. Play around until you get why they work—it’s like figuring out a new toy.
- Practice a Little Every Day: Try a coding puzzle or two whenever you’ve got a minute. Sites like LeetCode or HackerRank are packed with challenges—start small and build up.
- Make It Real: Code something yourself, like a list that adds and deletes things. Seeing it work is way more fun than just reading about it.
- Grab Some Help: Check out videos or tutorials online—tons are free and explain things with pictures. Watching an algorithm move step-by-step can click everything into place.
- Chat With Friends: Stuck? Talk it out with other learners online or in person. Someone else’s trick might be just what you need.
- Take It Easy: Some days, it’ll feel hard. That’s fine—step back, try again later, and cheer yourself on when you get it. Every little win counts.
Think of DSA like building a LEGO set. At first, it’s a pile of pieces, but stick with it, and soon you’ve got a spaceship. Keep going, mess up, laugh it off, and you’ll be amazed at what you can do.
To become a proficient coder and eventually land a career, you need to get a good grasp of Data Structures & Algorithms. While this is typically covered in your Data Structures class in college, if you didn't have the opportunity or need to refresh your knowledge, here's a highly-rated Coursera course from the University of Michigan with a 4.9 rating:
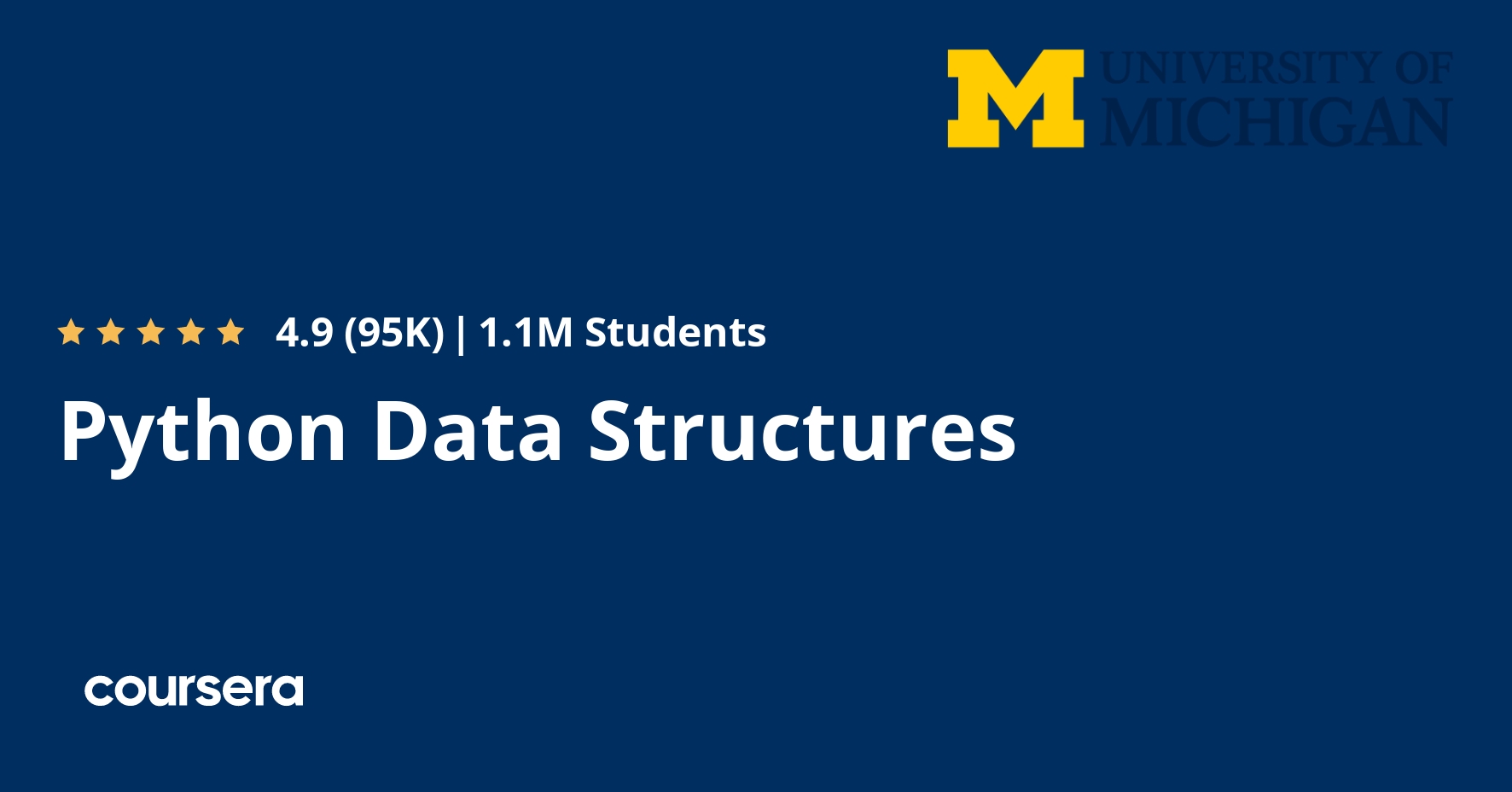